linked-list-cycle
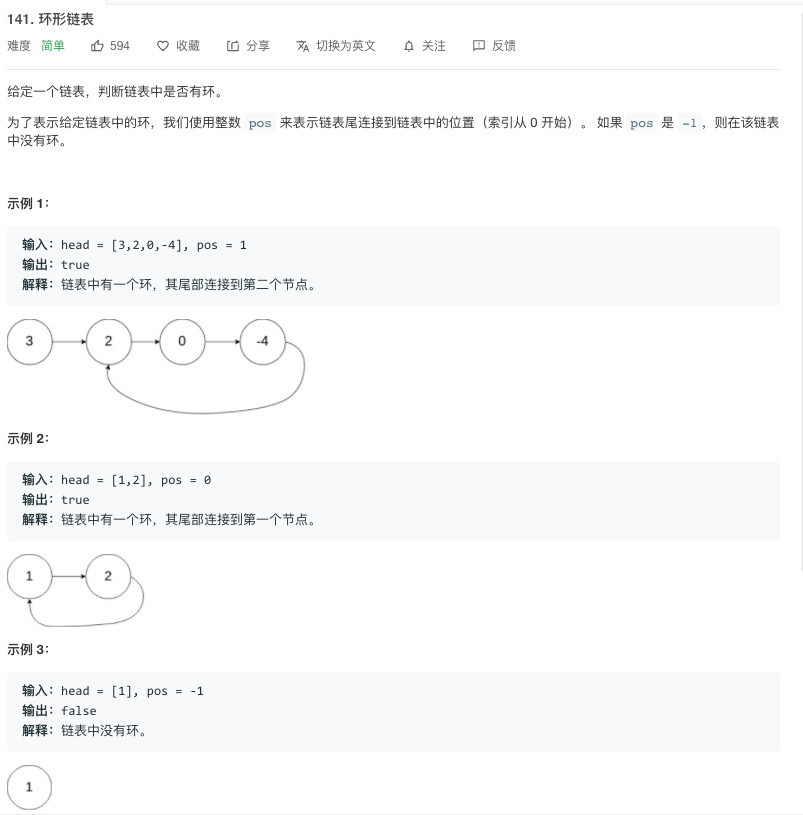
Method 1:
class Solution(object):
def hasCycle(self, head):
slow = fast = head
while fast and fast.next:
fast = fast.next.next
slow = slow.next
if slow == fast:
return True
return False
解析:快慢指针的方式,快指针一次走两步,慢指针一次走一次,如果在循环中出现了快慢指针相同,则有环。如果循环中一直没有相遇,则没有环。
Method 2:
class Solution(object):
def hasCycle(self, head):
"""
:type head: ListNode
:rtype: bool
"""
while head and head.val != None:
head.val, head = None, head.next
return head != None
解析:一个循环,一直遍历下一个指针,如果最后一个的next为空了则说明没有环,如果永远下一个不为空,则表示存在环